1.登陆拦截器
package com.dzqc.yx.interceptor;
import org.springframework.web.servlet.HandlerInterceptor;
import org.springframework.web.servlet.ModelAndView;
import com.alibaba.fastjson.JSONObject;
import com.dzqc.yx.util.AjaxResult;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.ArrayList;
import java.util.List;
/**
* 登录拦截器
* @author Boost
*/
public class LoginInterceptor implements HandlerInterceptor {
/**
* 开始进入地址请求拦截
*/
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler)
throws Exception {
HttpSession session = request.getSession();
if(session.getAttribute("user") != null){
return true;
}else{
String jsonObjectStr = JSONObject.toJSONString(new AjaxResult(1186,false,"login_error",null));
returnJson(response,jsonObjectStr);
return false;
}
}
/**
* 认证失败返回json数据
* @param response
* @param json
* @throws Exception
*/
@SuppressWarnings("unused")
private void returnJson(HttpServletResponse response, String json) throws Exception{
PrintWriter writer = null;
response.setCharacterEncoding("UTF-8");
response.setContentType("text/html; charset=utf-8");
try {
writer = response.getWriter();
writer.print(json);
} catch (IOException e) {
} finally {
if (writer != null)
writer.close();
}
}
/**
* 处理请求完成后视图渲染之前的处理操作
*/
@Override
public void postHandle(HttpServletRequest request, HttpServletResponse response, Object handler,
ModelAndView modelAndView) throws Exception {
}
/**
* 视图渲染之后的操作
*/
@Override
public void afterCompletion(HttpServletRequest request, HttpServletResponse response, Object handler, Exception ex)
throws Exception {
}
/**
* 定义排除拦截URL
* @return
*/
public static List<String> getUrl(){
List<String> url = new ArrayList<String>();
url.add("/tologin"); //登录页
url.add("/login/in"); //登录action URL
return url;
}
}
在preHandle方法的else语句块中也可以进行从定向到登陆页面,例
response.sendRedirect("/admin/login"); //未登录,跳转到登录页
2.启用拦截器
package com.dzqc.yx.interceptor;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.InterceptorRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
/***
* 注册拦截器
*/
@Configuration
public class WebappAdapter implements WebMvcConfigurer {
/**
* 注册拦截器
*/
@Override
public void addInterceptors(InterceptorRegistry registry) {
registry.addInterceptor(new LoginInterceptor()).addPathPatterns("/**").excludePathPatterns(LoginInterceptor.getUrl());
WebMvcConfigurer.super.addInterceptors(registry);
}
}
未登录时返回
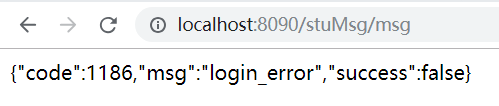