springmvc统一返回异常信息
当服务器代码为:
@RequestMapping("/res")
public MyAjaxResult res(@RequestParam(name="a",required=true)Integer a) {
a=1/0;
return MyAjaxResult.fail("ok", 100);
}
服务器对参数进行限制
浏览器请求时如过没有参数a,或者参数a不是int类型,就会报如下异常
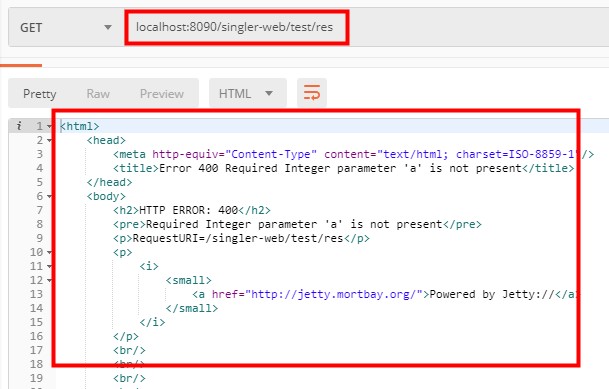
下面的配置就是把异常信息封装成json数据返回,而不在是返回一个页面
1.配置applicationContext-mvc.xml
<context:component-scan base-package="com.dzqc.dz.*.controller" use-default-filters="false">
<context:include-filter type="annotation" expression="org.springframework.stereotype.Controller" />
<context:include-filter type="annotation" expression="org.springframework.web.bind.annotation.ControllerAdvice" />
</context:component-scan>
2.异常拦截处理
package com.dzqc.dz.common.controller;
import java.io.IOException;
import org.springframework.beans.ConversionNotSupportedException;
import org.springframework.beans.TypeMismatchException;
import org.springframework.http.converter.HttpMessageNotReadableException;
import org.springframework.http.converter.HttpMessageNotWritableException;
import org.springframework.web.HttpMediaTypeNotAcceptableException;
import org.springframework.web.HttpRequestMethodNotSupportedException;
import org.springframework.web.bind.MissingServletRequestParameterException;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.ResponseBody;
import com.dzqc.dz.common.util.MyAjaxResult;
/**
* 异常增强,以JSON的形式返回给客服端
* 异常增强类型:NullPointerException,RunTimeException,ClassCastException,
NoSuchMethodException,IOException,IndexOutOfBoundsException
以及springmvc自定义异常等,如下:
SpringMVC自定义异常对应的status code
Exception HTTP Status Code
ConversionNotSupportedException 500 (Internal Server Error)
HttpMessageNotWritableException 500 (Internal Server Error)
HttpMediaTypeNotSupportedException 415 (Unsupported Media Type)
HttpMediaTypeNotAcceptableException 406 (Not Acceptable)
HttpRequestMethodNotSupportedException 405 (Method Not Allowed)
NoSuchRequestHandlingMethodException 404 (Not Found)
TypeMismatchException 400 (Bad Request)
HttpMessageNotReadableException 400 (Bad Request)
MissingServletRequestParameterException 400 (Bad Request)
*/
@ControllerAdvice
public class RestExceptionHandler{
//运行时异常
@ExceptionHandler(RuntimeException.class)
@ResponseBody
public MyAjaxResult runtimeExceptionHandler(RuntimeException ex) {
return MyAjaxResult.fail(ex.getClass().getName()+":"+ex.getMessage());
}
//空指针异常
@ExceptionHandler(NullPointerException.class)
@ResponseBody
public MyAjaxResult nullPointerExceptionHandler(NullPointerException ex) {
ex.printStackTrace();
return MyAjaxResult.fail(ex.getClass().getName()+":"+ex.getMessage());
}
//类型转换异常
@ExceptionHandler(ClassCastException.class)
@ResponseBody
public MyAjaxResult classCastExceptionHandler(ClassCastException ex) {
ex.printStackTrace();
return MyAjaxResult.fail(ex.getClass().getName()+":"+ex.getMessage());
}
//IO异常
@ExceptionHandler(IOException.class)
@ResponseBody
public MyAjaxResult iOExceptionHandler(IOException ex) {
ex.printStackTrace();
return MyAjaxResult.fail(ex.getClass().getName()+":"+ex.getMessage());
}
//未知方法异常
@ExceptionHandler(NoSuchMethodException.class)
@ResponseBody
public MyAjaxResult noSuchMethodExceptionHandler(NoSuchMethodException ex) {
ex.printStackTrace();
return MyAjaxResult.fail(ex.getClass().getName()+":"+ex.getMessage());
}
//数组下表
@ExceptionHandler(IndexOutOfBoundsException.class)
@ResponseBody
public MyAjaxResult indexOutOfBoundsExceptionHandler(IndexOutOfBoundsException ex) {
ex.printStackTrace();
return MyAjaxResult.fail(ex.getClass().getName()+":"+ex.getMessage());
}
//400错误
@ExceptionHandler({HttpMessageNotReadableException.class})
@ResponseBody
public MyAjaxResult requestNotReadable(HttpMessageNotReadableException ex){
ex.printStackTrace();
return MyAjaxResult.fail(ex.getClass().getName()+":"+ex.getMessage(),400);
}
//400错误
@ExceptionHandler({TypeMismatchException.class})
@ResponseBody
public MyAjaxResult requestTypeMismatch(TypeMismatchException ex){
ex.printStackTrace();
return MyAjaxResult.fail(ex.getClass().getName()+":"+ex.getMessage(),400);
}
//400错误
@ExceptionHandler({MissingServletRequestParameterException.class})
@ResponseBody
public MyAjaxResult requestMissingServletRequest(MissingServletRequestParameterException ex){
return MyAjaxResult.fail(ex.getClass().getName()+":"+ex.getMessage(),400);
}
//405错误
@ExceptionHandler({HttpRequestMethodNotSupportedException.class})
@ResponseBody
public MyAjaxResult request405(HttpRequestMethodNotSupportedException ex){
return MyAjaxResult.fail(ex.getClass().getName()+":"+ex.getMessage(),405);
}
//406错误
@ExceptionHandler({HttpMediaTypeNotAcceptableException.class})
@ResponseBody
public MyAjaxResult request406(HttpMediaTypeNotAcceptableException ex){
return MyAjaxResult.fail(ex.getClass().getName()+":"+ex.getMessage(),406);
}
//500错误
@ExceptionHandler({ConversionNotSupportedException.class,HttpMessageNotWritableException.class})
@ResponseBody
public MyAjaxResult server500(RuntimeException ex){
return MyAjaxResult.fail(ex.getClass().getName()+":"+ex.getMessage(),500);
}
}
如请求如下方法
@RequestMapping("/res")
public MyAjaxResult res(@RequestParam(name="a",required=true)Integer a) {
a=1/0;
return MyAjaxResult.fail("ok", 100);
}
请求为: localhost:8090/singler-web/test/res
返回结果:
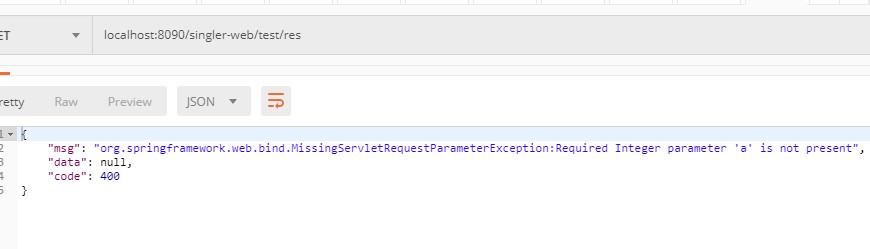
请求为:localhost:8090/singler-web/test/res?a=1
返回结果:
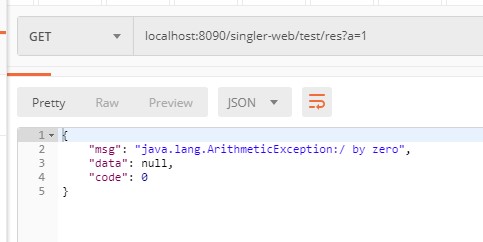
把res方法中的a=1/0去掉就会正常返回结果