springmvc启动时从数据库中初始化系统常量
设计的目标是,把项目的系统常量配置,放在数据库中,在项目初始化时从项目中获取配置信息,利用反射技术,把key-value对应的值自动封装进配置类。
1.例:数据库中的字段与实体类中的key对应,实现自动封装
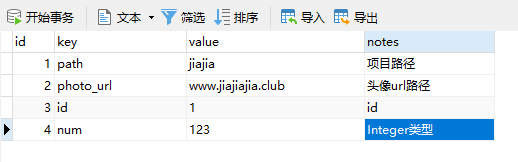
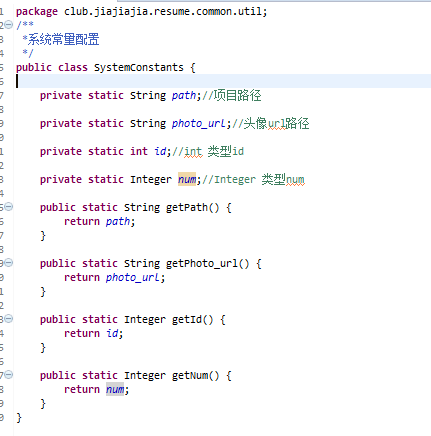
2.实体类:
package club.jiajiajia.resume.common.entity;
public class KeyValue {
private Integer id;
private String key;
private String value;
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getKey() {
return key;
}
public void setKey(String key) {
this.key = key;
}
public String getValue() {
return value;
}
public void setValue(String value) {
this.value = value;
}
}
3.系统配置类:
package club.jiajiajia.resume.common.util;
/**
*系统常量配置
*/
public class SystemConstants {
private static String path;//项目路径
private static String photo_url;//头像url路径
private static int id;//int 类型id
private static Integer num;//Integer 类型num
public static String getPath() {
return path;
}
public static String getPhoto_url() {
return photo_url;
}
public static Integer getId() {
return id;
}
public static Integer getNum() {
return num;
}
}
4.项目启动时执行controller,利用反射技术实现自动封装
package club.jiajiajia.resume.common.controller;
import java.lang.reflect.Field;
import java.util.List;
import javax.servlet.ServletContext;
import org.springframework.beans.factory.InitializingBean;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.context.ServletContextAware;
import club.jiajiajia.resume.common.dao.SystemMapper;
import club.jiajiajia.resume.common.entity.KeyValue;
import club.jiajiajia.resume.common.util.SystemConstants;
/**
* 从数据库中初始化参数
* @author Administrator
*/
public class SystemController implements InitializingBean, ServletContextAware {
@Autowired
private SystemMapper systemMapper;
/***
* 系统配置自动化封装
*/
@Override
public void setServletContext(ServletContext arg0){
List<KeyValue> sys=systemMapper.system();
Class<?> c=SystemConstants.class;
Field f=null;
for(KeyValue kv:sys){
if(kv.getValue()!=null&&!"".equals(kv.getValue())){
try {
f=c.getDeclaredField(kv.getKey());
f.setAccessible(true);
if(f.getType()==int.class||f.getType()==Integer.class){
f.set(SystemConstants.class,new Integer(kv.getValue()));
}else if(f.getType()==String.class){
f.set(SystemConstants.class,kv.getValue());
}
} catch (NoSuchFieldException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (SecurityException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (NumberFormatException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IllegalArgumentException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IllegalAccessException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
}
@Override
public void afterPropertiesSet() throws Exception {
// TODO Auto-generated method stub
}
}
5.访问数据库:
package club.jiajiajia.resume.common.dao;
import java.util.List;
import club.jiajiajia.resume.common.entity.KeyValue;
public interface SystemMapper {
public List<KeyValue> system();
}
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd" >
<mapper namespace="club.jiajiajia.resume.common.dao.SystemMapper" >
<select id="system" resultType="club.jiajiajia.resume.common.entity.KeyValue">
SELECT sc.id,sc.key,sc.value from system_config sc
</select>
</mapper>
6.applicationContext.xml配置:放进ioc容器中
<bean class="club.jiajiajia.resume.common.controller.SystemController"></bean>
这样系统启动的时候就会初始化执行SystemController类,自动封装属性
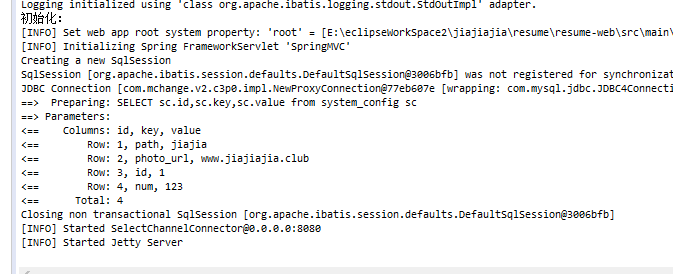
但注意:数据库中的value字段的值一定要与属性的类型对应,不可能int类型的属性放一个字符串的值。