基于 双向链表结构
直接代码:
package threadTest.test6;
public class Node<E> {
private E e;
private Node<E> prve;
private Node<E> next;
public Node(E e) {
super();
this.e = e;
}
public E getE() {
return e;
}
public void setE(E e) {
this.e = e;
}
public Node<E> getPrve() {
return prve;
}
public void setPrve(Node<E> prve) {
this.prve = prve;
}
public Node() {
super();
// TODO Auto-generated constructor stub
}
public Node<E> getNext() {
return next;
}
public void setNext(Node<E> next) {
this.next = next;
}
@Override
protected void finalize() throws Throwable {
System.out.println("当前对象被释放:"+e);
}
}
package threadTest.test6;
public class Queue<E> {
private Node<E> head;//队首
private Node<E> tail;//队尾
private Integer size;//队列长度
private Integer s;//当前队列长度
/**
* 定义队列最大长度 size
* @param size
*/
public Queue(Integer size) {
super();
head = new Node<E>();
tail = new Node<E>();
head.setNext(tail);
head.setPrve(null);
tail.setNext(null);
tail.setPrve(head);
this.size = size;
this.s=0;
}
/**
* 默认队列长度为:Integer.MAX_VALUE
*/
public Queue() {
super();
head = new Node<E>();
tail = new Node<E>();
head.setNext(tail);
head.setPrve(null);
tail.setNext(null);
tail.setPrve(head);
this.size=Integer.MAX_VALUE;
this.s=0;
}
/**
* 入队操作
* @param e
*/
public void push(E e) {
if(this.s>=this.size) {
System.err.println("队列已满,入队失败");
return;
}
Node<E> n=new Node<E>(e);
n.setPrve(tail.getPrve());
n.setNext(tail);
tail.getPrve().setNext(n);
tail.setPrve(n);
this.s++;
}
/**
* 出队操作
* @return
*/
public E pop() {
if(this.s<=0) {
System.err.println("空队列");
return null;
}
Node<E> n=head.getNext();
n.getNext().setPrve(head);
head.setNext(n.getNext());
s--;
return n.getE();
}
@Override
public String toString() {
Node<E> no=head.getNext();
while(no!=tail) {
System.out.println(no.getE());
no=no.getNext();
}
return "";
}
}
package threadTest.test6;
public class TestMain {
public static void main(String[] args) {
Queue<Integer> q=new Queue<>(3);
q.push(1);
q.push(2);
q.push(3);
q.push(4);
q.push(5);
q.push(6);
System.out.println(q.pop());
System.out.println(q.pop());
System.out.println(q.pop());
System.out.println(q.pop());
}
}
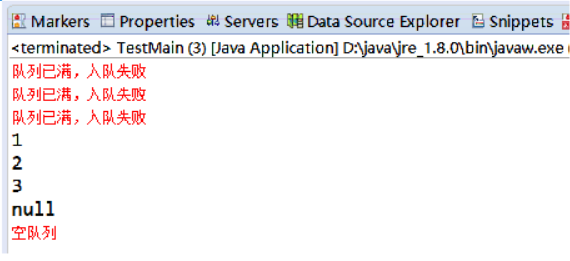